やりたいこと
- Teamsで以下のようにデータを表示したい
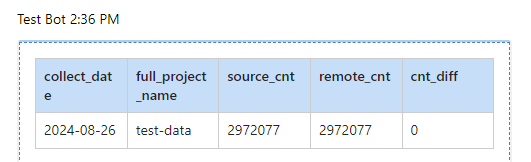
- こちらのサイトでadaptive cardをpreviewできる
- 以下のjsonで、テーブル型のデータをadaptive cardで表示できる
{
"$schema": "http://adaptivecards.io/schemas/adaptive-card.json",
"type": "AdaptiveCard",
"version": "1.5",
"body": [
{
"type": "Table",
"columns": [
{
"width": 1
},
{
"width": 1
},
{
"width": 1
},
{
"width": 1
},
{
"width": 1
}
],
"rows": [
{
"type": "TableRow",
"cells": [
{
"type": "TableCell",
"items": [
{
"type": "TextBlock",
"text": "collect_date",
"weight": "Bolder",
"wrap": true
}
]
},
{
"type": "TableCell",
"items": [
{
"type": "TextBlock",
"text": "full_project_name",
"weight": "Bolder",
"wrap": true
}
]
},
{
"type": "TableCell",
"items": [
{
"type": "TextBlock",
"text": "source_cnt",
"weight": "Bolder",
"wrap": true
}
]
},
{
"type": "TableCell",
"items": [
{
"type": "TextBlock",
"text": "remote_cnt",
"weight": "Bolder",
"wrap": true
}
]
},
{
"type": "TableCell",
"items": [
{
"type": "TextBlock",
"text": "cnt_diff",
"weight": "Bolder",
"wrap": true
}
]
}
],
"style": "accent"
},
{
"type": "TableRow",
"cells": [
{
"type": "TableCell",
"items": [
{
"type": "TextBlock",
"text": "2024-08-26",
"wrap": true
}
]
},
{
"type": "TableCell",
"items": [
{
"type": "TextBlock",
"text": "test-data",
"wrap": true
}
]
},
{
"type": "TableCell",
"items": [
{
"type": "TextBlock",
"text": "2972077",
"wrap": true
}
]
},
{
"type": "TableCell",
"items": [
{
"type": "TextBlock",
"text": "2972077",
"wrap": true
}
]
},
{
"type": "TableCell",
"items": [
{
"type": "TextBlock",
"text": "0",
"wrap": true
}
]
}
]
}
]
}
]
}
Python- こちらの関数で、DF⇒teamsのadaptive cardを生成できる
def build_card(df):
for col in ['source_cnt', 'remote_cnt', 'cnt_diff']:
df[col] = df[col].apply(lambda x: f"{x:,}" if isinstance(x, (int, float)) else x)
# build the header of table
headers = [
{
"type": "TableCell",
"items": [{"type": "TextBlock", "text": col, "weight": "Bolder", "wrap": True}]
} for col in df.columns
]
# build rows of table
rows = []
for _, row in df.iterrows():
row_cells = [
{
"type": "TableCell",
"items": [{"type": "TextBlock", "text": str(value), "wrap": True}]
} for value in row
]
rows.append({"type": "TableRow", "cells": row_cells})
# build the json of adaptive card
adaptive_card = {
"$schema": "http://adaptivecards.io/schemas/adaptive-card.json",
"type": "AdaptiveCard",
"version": "1.3",
"body": [
{
"type": "Table",
"columns": [{"width": 1} for _ in df.columns], # length
"rows": [
{"type": "TableRow", "cells": headers, "style": "accent"}, # headers
*rows # rows
]
}
]
}
# build the message
message = {
"type": "message",
"attachments": [
{
"contentType": "application/vnd.microsoft.card.adaptive",
"content": adaptive_card
}
]
}
return message
Python- 以下のコードでteamsのwebhookをcall
message = build_card(df_2),
response = requests.post(
url=webhook_url_failed,
data=json.dumps(message),
headers={'Content-Type': 'application/json'}
)
Python